Servlet 3 web.xml Example Configuration
A servlet is a Java class that extends the javax.servlet.Servlet
interface. To handle HTTP request you need to extend the javax.servlet.http.HttpServlet
abstraction. Servlets interact with Web clients via a request/response paradigm implemented by the servlet container. In the following tutorial we will show you how to create a servlet 3 annotation example configuration.
Project structure
+--src
| +--main
| +--java
| +--com
| +--memorynotfound
| |--ExampleHttpServlet.java
| |--resources
| +--webapp
| +--WEB-INF
| |--web.xml
pom.xml
Maven Dependency
Every Servlet Container/Application Server has built in servlet support so you need to set the dependency on provided
. So Maven does not include the library in the final built artifact.
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Defining our Servlet
The HttpServlet abstract subclass adds additional methods beyond the basic Servlet interface that are automatically called by the service method in the HttpServlet class to aid in processing HTTP-based requests. These methods are
doGet
for handling HTTP GET requestsdoPost
for handling HTTP POST requestsdoPut
for handling HTTP PUT requestsdoDelete
for handling HTTP DELETE requestsdoHead
for handling HTTP HEAD requestsdoOptions
for handling HTTP OPTIONS requestsdoTrace
for handling HTTP TRACE requests
Typically when developing HTTP-based servlets, a Servlet Developer will only concern himself with the doGet and doPost methods. The other methods are considered to be methods for use by programmers very familiar with HTTP programming.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
public class AnnotationServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter out = resp.getWriter();
out.write("Servlet 3 web.xml example configuration");
}
}
Servlet 3 web.xml Configuration
Instead of using Servlet 3 annotation configuration, when using a deployment descriptor you must register your servlet using the <servlet>
tag with a required <servlet-name>
and <servlet-class>
tag which must include the full path of the servlet class which you want to map. To map this servlet to a specific URL you need to add a <servlet-mapping>
with the same <servlet-name>
and a <url-pattern>
to map your servlet to a url pattern.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<servlet>
<servlet-name>Example-Servlet</servlet-name>
<servlet-class>com.memorynotfound.ExampleHttpServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Example-Servlet</servlet-name>
<url-pattern>/example</url-pattern>
</servlet-mapping>
</web-app>
Demo
URL: http://localhost:8080/servlet/example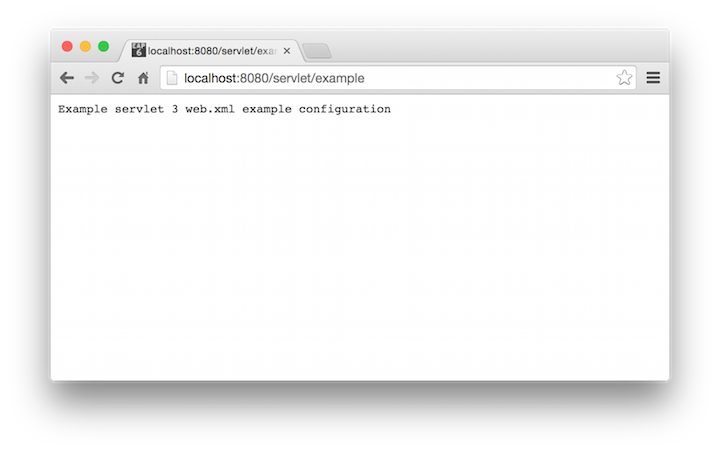