Servlet Attributes request session and application scope
In this tutorial we will talk about servlet attributes. Attributes can be objects not just strings and are used to pass data between requests. For a web application it is essential to pass data between different servlets. This way we can collect data from a form and save it to our database for example. There are three different scopes: request scope, session scope and application scope.
Project structure
+--src
| +--main
| +--java
| +--com
| +--memorynotfound
| |--GetAttributesServlet.java
| |--SetAttributesServlet.java
| |--resources
| |--webapp
pom.xml
Maven Dependency
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
Setting Servlet attributes: applicationScope, sessionScope, requestScope
Attributes can contain any object not just strings. And are used to pass data between requests. There are three different scopes of servlet attributes.
- Request Scope: are most useful when processing the results of a submitted form. With request scope you are also guaranteed that no other request will be able to affect your objects in that request scope while your request is being handled. This scope has the shortest lifspan.
- Session Scope: is associated with a user. When a user visits your web application, a session is created by the web container. So the session’s lifespan lives as long as the user interacts with your application or when
session.invalidate()
is called. - Application Scope: or global scope is associated with your web application. This scope lives as long as the web application is deployed.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
@WebServlet("/set-attributes")
public class SetAttributesServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// set application scoped attribute
req.getServletContext().setAttribute("name", "application scoped attribute");
// set session scoped attribute
HttpSession session = req.getSession();
session.setAttribute("name", "session scoped attribute");
// set request scoped attribute
req.setAttribute("name", "request scoped attribute");
// send redirect to other servlet
req.getRequestDispatcher("get-attributes").forward(req, resp);
}
}
Getting Servlet attributes: applicationScope, sessionScope, requestScope
Here is an example of how you can get servlet attributes.
package com.memorynotfound;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/get-attributes")
public class GetAttributesServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
// get application scoped attribute
String applicationScope = (String)req.getServletContext().getAttribute("a");
// get session scoped attribute
HttpSession session = req.getSession();
String sessionScope = (String)session.getAttribute("b");
// get request scoped attribute
String requestScope = (String)req.getAttribute("c");
// print response
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
out.write("<html><body>");
out.write("<h2>Servlet attributes example: applicationScope, sessionScope and requestScope</h2>");
out.write("<p>applicationScope: " + applicationScope + "</p>");
out.write("<p>sessionScope: " + sessionScope + "</p>");
out.write("<p>requestScope: " + requestScope + "</p>");
}
}
Demo
Getting Servlet attributes without setting
URL: http://localhost:8080/servlet-attributes/get-attributes
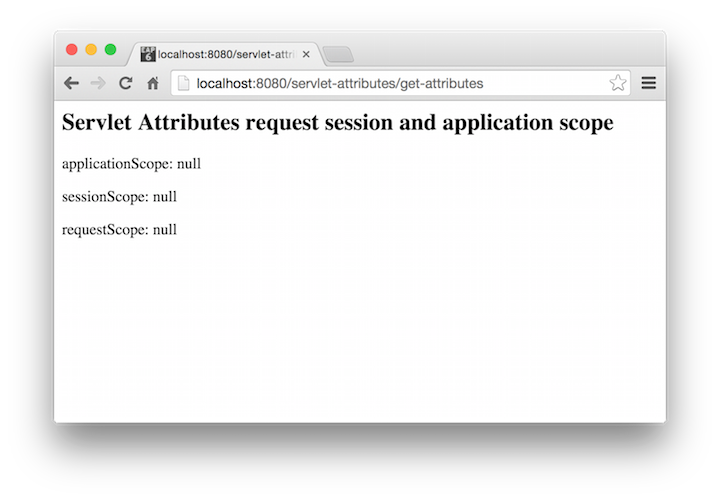
Setting Servlet attributes with forward
URL: http://localhost:8080/servlet-attributes/set-attributes
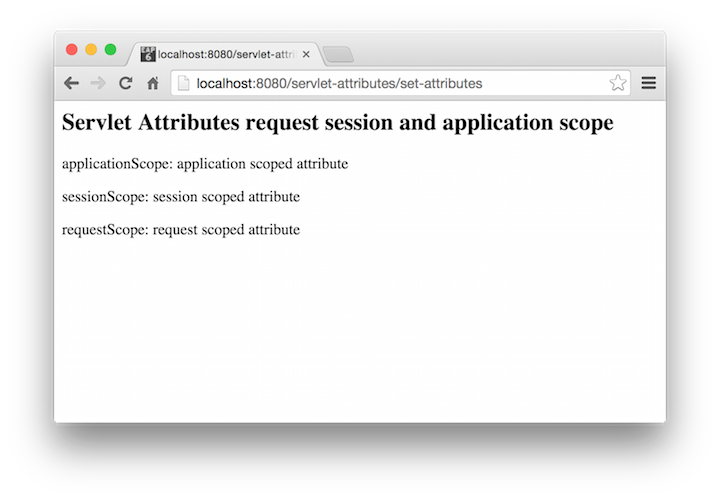
Refresh get-attributes
URL: http://localhost:8080/servlet-attributes/get-attributes
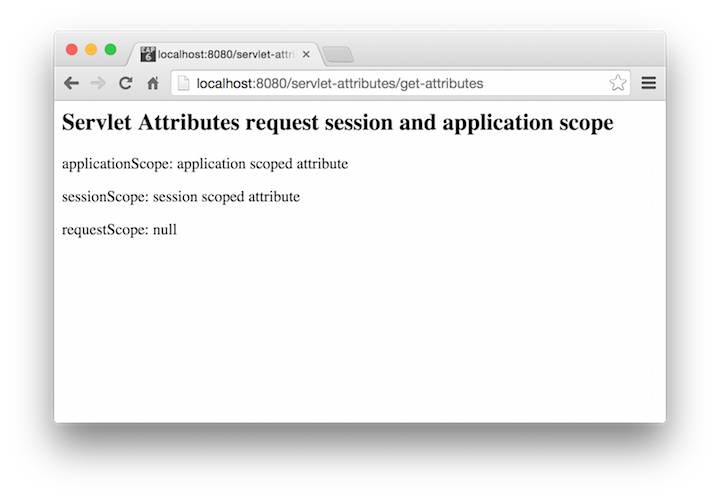
Java Servlet is really important concept , I am searching for such type of website all the basic concepts are well explained , Many thanks for sharing this.