Servlet Basic Authentication Annotation Configuration
This tutorial explains how to configure Servlet Basic Authentication annotation configuration. If you are planning on using basic authentication you must be aware that if you use plain HTTP requests your username and password can be intercepted by monitoring network communication, so I strongly recommend using HTTP with SSL (HTTPS). This way your data wil be encrypted. With Basic authentication, your username and password automatically get Base64 encoded which do not mean that it is safe. Base64 encoding can be easily decrypted. In the following example we will guide through the steps in how to configure a servlet with base authentication using annotation configuration.
Configure Username/password
This example uses tomcat-users.xml file to register a username/password combination with the appropriate row. This file is located at ../tomcat-home/conf/tomcat-users.xml
.
<?xml version='1.0' encoding='utf-8'?>
<tomcat-users xmlns="http://tomcat.apache.org/xml"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://tomcat.apache.org/xml tomcat-users.xsd"
version="1.0">
<user username="tomcat" password="tomcat" roles="secured"/>
</tomcat-users>
Secured Servlet
Using annotations to configure our servlet can completely eliminate the use of a servlet descriptor. However there still are some configuration which are not currently available using annotations. We’ll get to that later. First lets see what’s happening here. We can register our servlet using the @WebServlet
annotation. Next we can secure the servlet using the @ServletSecurity
annotation, you can configure which roles are allowed and specify which HttpMethods are restricted.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.HttpConstraint;
import javax.servlet.annotation.HttpMethodConstraint;
import javax.servlet.annotation.ServletSecurity;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/secured")
@ServletSecurity(
value = @HttpConstraint(
rolesAllowed = {
"secured"
}),
httpMethodConstraints = {
@HttpMethodConstraint(value = "GET", rolesAllowed = {
"secured"
}),
@HttpMethodConstraint(value = "POST")
})
public class SecuredServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter out = resp.getWriter();
out.write("Servlet basic authentication annotation configuration: secured servlet");
}
}
Public Servlet
Next as an example we will create a servlet that will be publicly available without security.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/public")
public class PublicServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter out = resp.getWriter();
out.write("Servlet basic authentication annotation configuration: public servlet");
}
}
Servlet Basic Authentication XML Servlet Descriptor
As we said earlier: we cannot completely remove our servlet descriptor because if we want to get the popup from the browser we still need to configure the authentication method used for authenticating. If however you do not need this popup and you’ll pass the authorization in the header then ofcourse you could just delete the deployment descriptor from your project.
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1">
<login-config>
<auth-method>BASIC</auth-method>
<realm-name>default</realm-name>
</login-config>
</web-app>
Demo
URL: http://localhost:8080/servlet-basic-authentication-xml/public
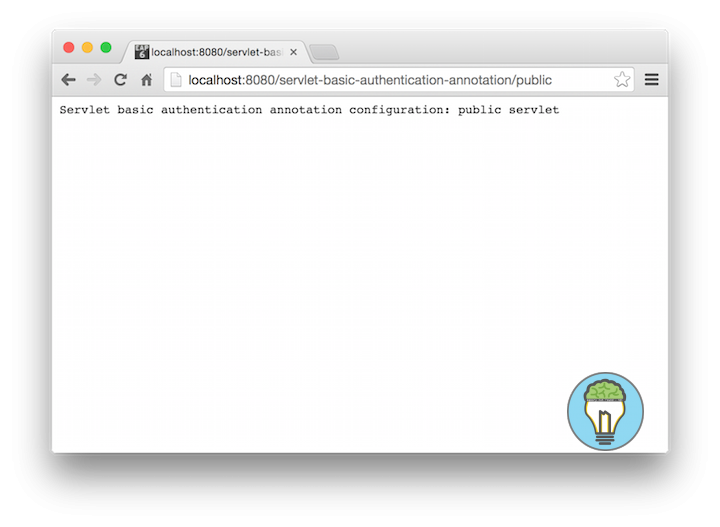
URL: http://localhost:8080/servlet-basic-authentication-xml/secured login popup
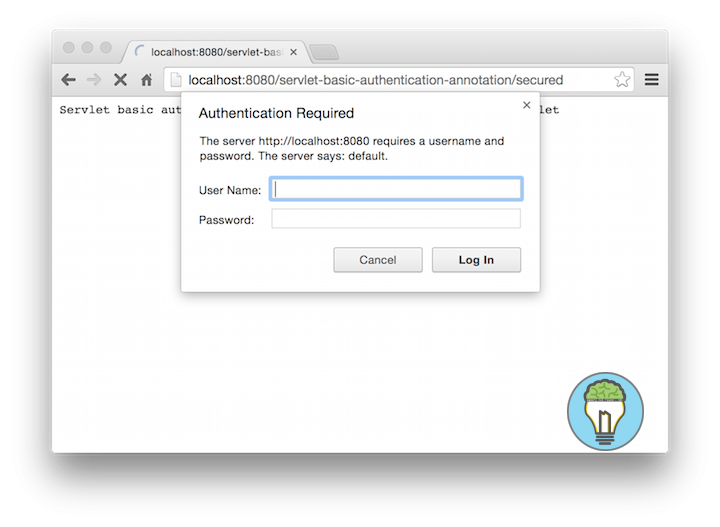
URL: http://localhost:8080/servlet-basic-authentication-xml/secured access denied
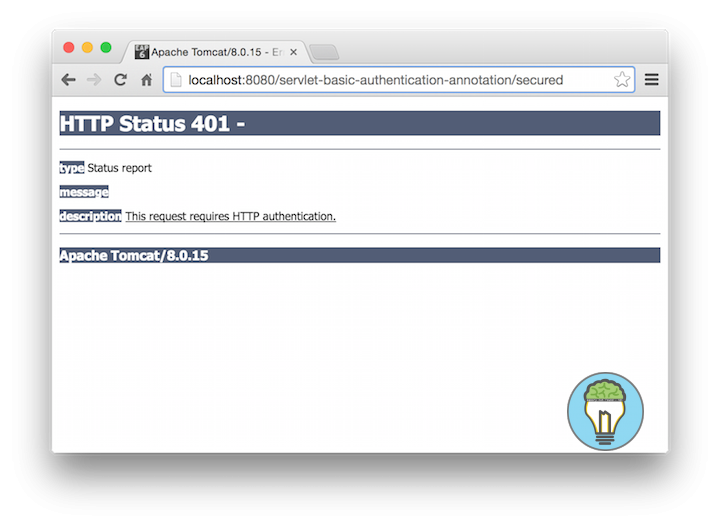
URL: http://localhost:8080/servlet-basic-authentication-xml/secured logged in
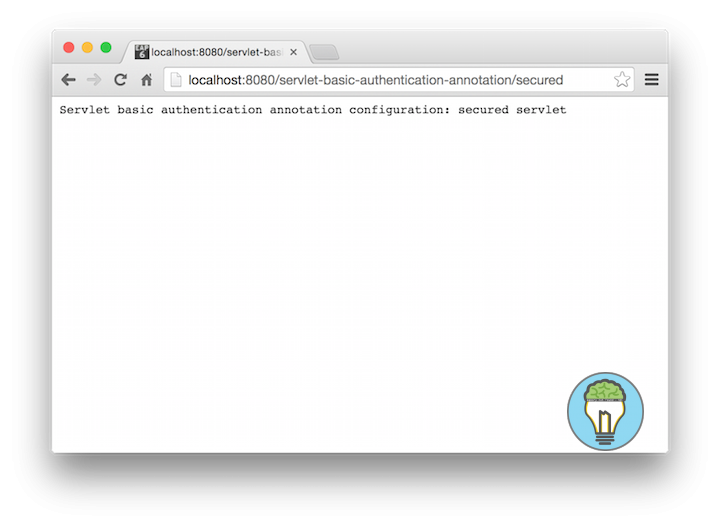