Servlet forward HTTP Request Example
In this example we will forward an HTTP Request from a servlet to another servlet. The forward method of the RequestDispatcher
will forward a request from a servlet to another resource. This can be either another servlet, JSP file or HTML file on the server. The forward should be called before anything is written to the ServletOutputStream
. If the response already has been committed, this method throws an IllegalStateException
indicating that the response has already been written for that request.
Servlet Forward HTTP Request
The forward method of the dispatcher sets the request type to DispatcherType.FORWARD
.
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/forward")
public class ForwardServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.getRequestDispatcher("/landing").forward(req, resp);
}
}
Servlet Forwarded HTTP Request
package com.memorynotfound;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@WebServlet("/landing")
public class LandingServlet extends HttpServlet{
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
PrintWriter out = resp.getWriter();
out.write("Servlet forward HTTP Request Example");
}
}
Demo
URL: localhost:8080/servlet-forward/forward
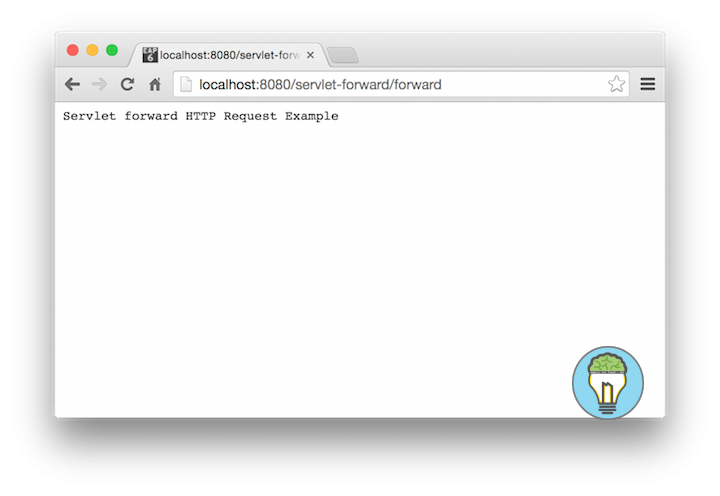