Spring Boot Ehcache 2 Caching Example Configuration
Ehcache is an open source, standards-based cache that boosts performance, offloads your database, and simplifies scalability. It’s the most widely-used Java-based cache because it’s robust, proven, full-featured, and integrates with other popular libraries and frameworks. Ehcache scales from in-process caching, all the way to mixed in-process/out-of-process deployments with terabyte-sized caches.
In this tutorial we demonstrate how to implement Ehcache 2 Caching configured using Spring Boot.
Project Structure
Our project structure looks like this.
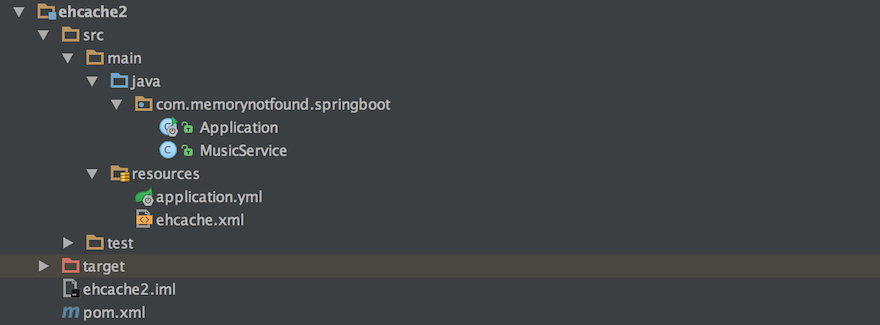
Maven Dependencies
We use Apache Maven to manage our project dependencies. To start, add the following dependencies to your project. When using ehcache
add the net.sf.ehcache:ehcache
dependency.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.memorynotfound.springboot.caching</groupId>
<artifactId>ehcache2</artifactId>
<version>1.0.0-SNAPSHOT</version>
<url>https://memorynotfound.com</url>
<name>Spring Boot - ${project.artifactId}</name>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.4.RELEASE</version>
</parent>
<dependencies>
<!-- Spring Framework Caching Support -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Ehcache 2 Caching Configuration
In this example configuration we’re creating a simple in memory cache. Create a ehcache.xml
file in the src/main/resources
folder. When the in memory cache isn’t sufficient for you, don’t worry. Ehcache has a lot of options you can tweak. For more advanced configurations, read the documentation.
<?xml version="1.0" encoding="UTF-8"?>
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://www.ehcache.org/ehcache.xsd"
updateCheck="true"
monitoring="autodetect"
dynamicConfig="true">
<cache name="instruments"
maxElementsInMemory="100"
eternal="false"
overflowToDisk="false"
timeToLiveSeconds="300"
timeToIdleSeconds="0"
memoryStoreEvictionPolicy="LFU"
transactionalMode="off">
</cache>
</ehcache>
Configure Caching Properties
We can configure the location of the ehcache.xml
in spring-boot by setting the following configuration property in the application.yml
file.
# application.yml
spring:
cache:
ehcache:
config: classpath:ehcache.xml
The equivalent application.properties
file.
# application.properties
spring.cache.ehcache.config=classpath:ehcache.xml
Ehcache 2 Caching Service
By annotating the play
method using the @Cacheable
annotation the result of the subsequent invocations will be cached. The @CacheEvict(allEntries=true)
clears all the entries from the cache. The @CacheConfig(cachenames="instruments")
registers every method annotated with the spring framework caching annotations with the specified cache.
package com.memorynotfound.springboot;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.cache.annotation.*;
import org.springframework.stereotype.Service;
@Service
@CacheConfig(cacheNames = "instruments")
public class MusicService {
private static Logger log = LoggerFactory.getLogger(MusicService.class);
@CacheEvict(allEntries = true)
public void clearCache(){}
@Cacheable(condition = "#instrument.equals('trombone')")
public String play(String instrument) {
log.info("Executing: " + this.getClass().getSimpleName() + ".play(\"" + instrument + "\");");
return "paying " + instrument + "!";
}
}
Bootstrap Ehcache 2 Caching Application
We can simply enable the caching by annotating the class with @EnableCaching
.
package com.memorynotfound.springboot;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.EnableCaching;
@EnableCaching
@SpringBootApplication
public class Application implements CommandLineRunner {
private static Logger log = LoggerFactory.getLogger(Application.class);
@Autowired
private MusicService musicService;
@Autowired
private CacheManager cacheManager;
public static void main(String[] args) throws Exception {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) throws Exception {
log.info("Spring Boot Ehcache 2 Caching Example Configuration");
log.info("using cache manager: " + cacheManager.getClass().getName());
musicService.clearCache();
play("trombone");
play("guitar");
play("trombone");
play("bass");
play("trombone");
}
private void play(String instrument){
log.info("Calling: " + MusicService.class.getSimpleName() + ".play(\"" + instrument + "\");");
musicService.play(instrument);
}
}
Running Spring Boot Application
We can run the spring boot application using the following maven command.
mvn spring-boot:run
Console Output
The previous application prints the following output to the console.
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v1.5.4.RELEASE)
INFO - Spring Boot Ehcache 2 Caching Example Configuration
INFO - using cache manager: org.springframework.cache.ehcache.EhCacheCacheManager
INFO - Calling: MusicService.play("trombone");
INFO - Executing: MusicService.play("trombone");
INFO - Calling: MusicService.play("guitar");
INFO - Executing: MusicService.play("guitar");
INFO - Calling: MusicService.play("trombone");
INFO - Calling: MusicService.play("bass");
INFO - Executing: MusicService.play("bass");
INFO - Calling: MusicService.play("trombone");
References
- Spring Caching Abstraction Documentation
- Ehcache Official Website
- Ehcache.xml Documentation
- Ehcache API JavaDoc
- Ehcache Documentation
Nice article :) quick question how to get all list of cache entries for manually evict. Please let me know. Thanks in advance.
You can take a look at
net.sf.ehcache.CacheManager
.